3D Printed T-800 Endoskeleton Part 6
Arduino work
I created a small program on my Arduino Uno to flash the eyes randomly, like there is something wrong with it.
I dont like the standard Arduino IDE, so I went for arduino-cli instead. That way i can use whatever editor I want (I prefer vim).
I have a small raspberry pi computer that I use as a development machine when programming the arduino. It's good enough for smaller projects. For bigger projects I use my laptop or stationary.
This is what the sketch looks like, it's very basic, nothing fancy.
random-eyes.ino
int ledPinLeft = 13;
int ledPinRight = 12;
long randOn = 0;
long randOff = 0;
void setup()
{
randomSeed(analogRead (0));
pinMode(ledPinLeft, OUTPUT);
pinMode(ledPinRight, OUTPUT);
digitalWrite(ledPinRight, HIGH);
}
void flashLeft(int ms)
{
digitalWrite(ledPinLeft, HIGH);
delay(ms);
digitalWrite(ledPinLeft, LOW);
delay(ms);
}
void flashRight(int ms)
{
digitalWrite(ledPinRight, HIGH);
delay(ms);
digitalWrite(ledPinRight, LOW);
delay(ms);
digitalWrite(ledPinRight, HIGH);
}
void flashRandom()
{
randOn = random(600, 2500);
randOff = random(10, 200);
digitalWrite(ledPinLeft, HIGH);
delay(randOn);
digitalWrite(ledPinLeft, LOW);
delay(randOff);
}
void loop()
{
flashRandom();
flashLeft(60);
flashRight(30);
flashLeft(60);
}
Gallery
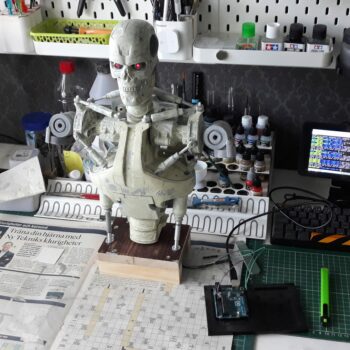